Last week, while running one of our functional test suites that relies on a specific environment variable, I forgot to set it—classic oversight. The result? An abrupt and unhelpful Python KeyError
, offering little insight into what went wrong.
That experience raised two important questions:
- What options do I have to access environment variables in Python?
- Which method is the most reliable?
Let’s explore both and see which one fits your use case best.
Accessing Environment Variables with os.environ[key]
The first method uses a dictionary-like object, os.environ
, which is pre-populated with all environment variables when the Python process starts. It’s a familiar and straightforward interface for directly retrieving values by their keys.
Let’s try it:
![For python environment variables with os.environ[key], do the following: export MY_VAR="test", python3 -i, import os and os.environ["MY_VAR"]. This should yield 'test'.](https://www.florianreinhard.de/wp-content/uploads/2021/12/Bildschirmfoto-2021-12-23-um-17.29.18-1024x272.png)
But what happens when we attempt to access a variable that hasn’t been set?
os.environ["UNSET_VAR"] # Raises KeyError
Unless we’ve wrapped it in a try-except
block, this call will immediately raise a KeyError
and stop your script. That’s exactly what happened during my test run.
Using os.getenv(key, default=None)
The second approach is more high-level and uses the os.getenv()
function, which accepts the environment variable name and an optional default value. It’s safer to use because it won’t throw an error if the variable is missing.
Let’s test it:
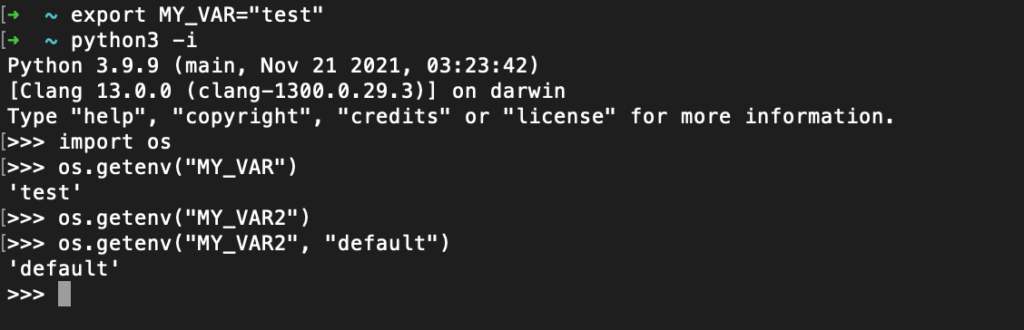
This method gives you more flexibility. If the environment variable isn’t set, os.getenv()
simply returns None
or a fallback default if provided. However, since the default return value is None
, this approach can introduce subtle bugs if not handled carefully—especially if None
is a valid value in your application logic. Always consider specifying an appropriate default value when using os.getenv()
.
Conclusion: Which One Should You Use?
We’ve explored both options—but which one is best for your use case?
Ultimately, the best choice depends on two key factors:
- Do you prefer low-level, strict access (with os.environ) or a more forgiving, high-level approach (os.getenv)?
- Do you want your code to fail fast with clear errors, or handle missing values more gracefully?
Both methods have their merits. Choose the one that aligns with your coding style and project needs.
That wraps up this quick dive into Python’s environment variable handling. Usually, I write more about Rust, like in my Cucumber Rust tutorial or my Rust module cheat sheet, but Python is my main language at work—so you can expect more content like this.
Also, with container technologies becoming increasingly central to my job, I’ll soon be sharing more tips—starting with a handy Docker ps
command trick. Or if you’re in the mood to keep coding, check out my Java threads tutorial next.
Happy holidays, and merry Christmas, Eeve!