Last week, I was about to execute a test run using one of our functional test suites that requires a certain environment variable – and I forgot to set it, whoops.. The answer was harsh and generic: A good ol‘ Python KeyError
. That put me up with two questions:
- What options do I have to get environment variables with Python and
- which one is the best?
Let’s start checking them out.
Introducing os.environ[key]
The first option is a simple dictionary that is prefilled with all environment variables when starting the Python process. This provides us with a simple-to-use interface that we already know and love.
Let’s try it out:
![For python environment variables with os.environ[key], do the following: export MY_VAR="test", python3 -i, import os and os.environ["MY_VAR"]. This should yield 'test'.](https://www.florianreinhard.de/wp-content/uploads/2021/12/Bildschirmfoto-2021-12-23-um-17.29.18-1024x272.png)
But what happens, when we query a variable that is not set? Then we hopefully prepared our try-except
Block, because that call is going down the river faster than sound. This happens because dictionaries throw generic KeyErrors
, when the queried key could not be found in the dict. That’s what happened in my test run.
os.getenv(key, default=None)
The second option follows a more higher-level approach by providing a function that takes our key in question and an optional default value. The usage is straight forward and it won’t crash as harshly as the first option does.
Let’s try it out:
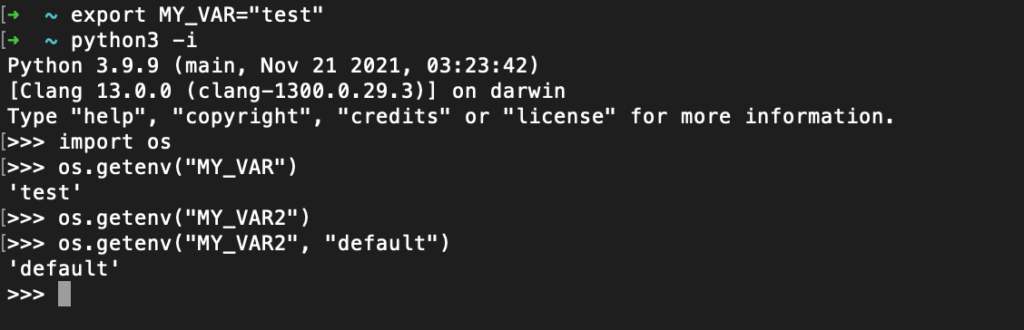
As we can see, we can now use any key we want; worst case is a result of None
. But the increased flexibility comes with a price: We have to take care of whatever the function returns. Even harder, due to the fact that the default defaults to None
(pun semi-intended), this solution is prone to hidden bugs. Therefore use it with care and set an appropriate default value if possible.
Conclusion: Which one works best for you?
We saw both options now, but which one works best (for you)? At the end of the day, it comes down to preference regarding two factors:
- Do you like your code to be more low-level or high-level?
- Do you want your fails fast or more controlled?
This is a decision you have to make, but once you have it, you find everything you need to get your environment variables with Python right here.
So long for the quick journey into my Python life. Usually I talk more about Rust as in my Cucumber Rust – tutorial and in this cheat sheet about Rust modules. But since Python is my main language on the job, there will definitely be more coming up soon. So if you are a friend of british comedy, feel free to stay tuned. Additionally, since container technologies are getting more and more important in my day job as well, I will write more about these techs, too, starting with this little docker ps – trick. Or, if you rather keep coding, check out my new Java threads tutorial.
Happy holidays and a merry Christmas Eeve!